Breadth First Search (BFS) and Depth First Search (DFS)
Graph Data Structure can be used to represent networks (like social network) or relationships between one element to another. So for example if the Graph below was representing a social networking site then you can say a Person 0 has Person 1 and Person 4 as connections. Person 1 in turn has Person 0,2,3 and 4 as its connections and so forth.
Breadth First Search(BFS) and Depth First Search(DFS) are two graph traversing algorithms. When traversing a graph there are two terminologies that are used, visit a vertex and explore a vertex. To visit a vertex simply means to go to that vertex. To explore a vertex means to find out what are the other vertices to which the current vertex is connected to.
Breadth First Search(BFS)
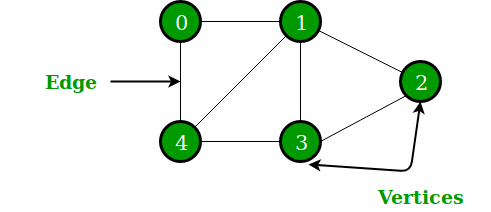
- If 0 is our starting vertex then in BFS we visit 0 and then explore the neighbors of 0 which are 1 and 4. So our set of vertices are {0, 1, 4}.
- Next we are visiting 1 (or 4) and explore neighbors of 1 which are 0,2,3,4. Now that 0 and 4 are already part of the set of vertices we will not include them ,so our set looks like this {0,1,4,2,3}
- Visit 2 and explore its neighbors. Its neighbors are 1 and 3. since we already explored 1 and 3 we skip these vertices {0,1,4,2,3}.
- Visit 3, neighbors are 1,2 and 4 - all of them are already visited so skip them. Our set still remains{0,1,4,2,3}.
- Visit 4, neighbors are 0,1 and 3 - all of them are already visited so skip them. Our set still remains {0,1,4,2,3}.
Implementation of BFS JavaScript:
const adjacencyList = new Map(); adjacencyList.set(0, new Set([1,4])); adjacencyList.set(1, new Set([0,4,2,3])); adjacencyList.set(2, new Set([1,3])); adjacencyList.set(3, new Set([1,4,2])); adjacencyList.set(4, new Set([3,0,1]));
const visit = console.log;
const bfs = (startNode) => { const visited = new Set(); const queue = []; queue.push(startNode); visited.add(startNode); while (queue.length > 0) { const currentNode = queue.shift(); visit(currentNode); for (let neighbor of adjacencyList.get(currentNode)) { if (!visited.has(neighbor)) { queue.push(neighbor); visited.add(neighbor); } } } };
Practical Application of BFS
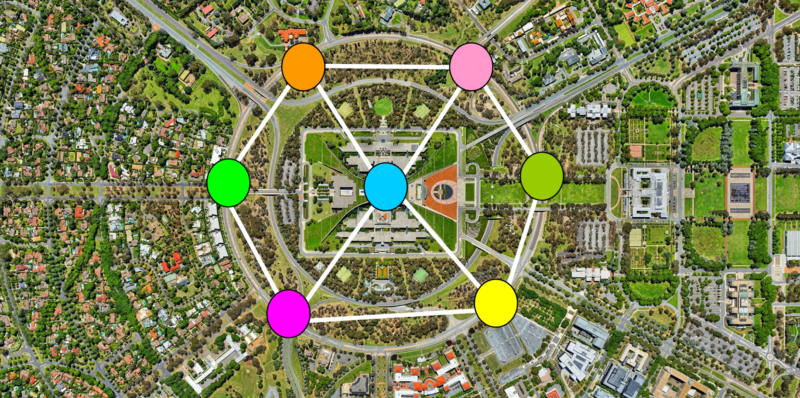
Depth First Search (DFS)
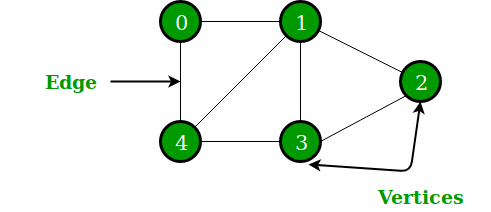
- If 0 is the starting vertex and we if we are traversing the top edges then we will visit {0,1,2}.
- Next we explore 2, whose neighbors are 1 and 3. So our set becomes {0,1,2,3}. Note that we skipped 1 since it is already in the set of vertices so we need not repeat it.
- Then we explore 1, whose neighbors 2,3 and 4. So our set becomes {0,1,2,3,4}.
- Then we explore 0, whose neighbors 1 and 4. Since we already have 1 and 4 in our set of vertices the final result looks like this {0,1,2,3,4}.
const adjacencyList = new Map(); adjacencyList.set(0, new Set([1,4])); adjacencyList.set(1, new Set([0,2,3,4])); adjacencyList.set(2, new Set([1,3])); adjacencyList.set(3, new Set([1,2,4])); adjacencyList.set(4, new Set([0,1,3])); const visit = console.log; const visited = new Set(); const dfs = (node) => { visit(node); visited.add(node); for (let neighbour of adjacencyList.get(node)) { if (!visited.has(neighbour)) { dfs(neighbour); } } };